1. IntelliJ IDEA와 Git Bash 연동
settings을 위해 ctrl + alt + s => terminal에서 세팅
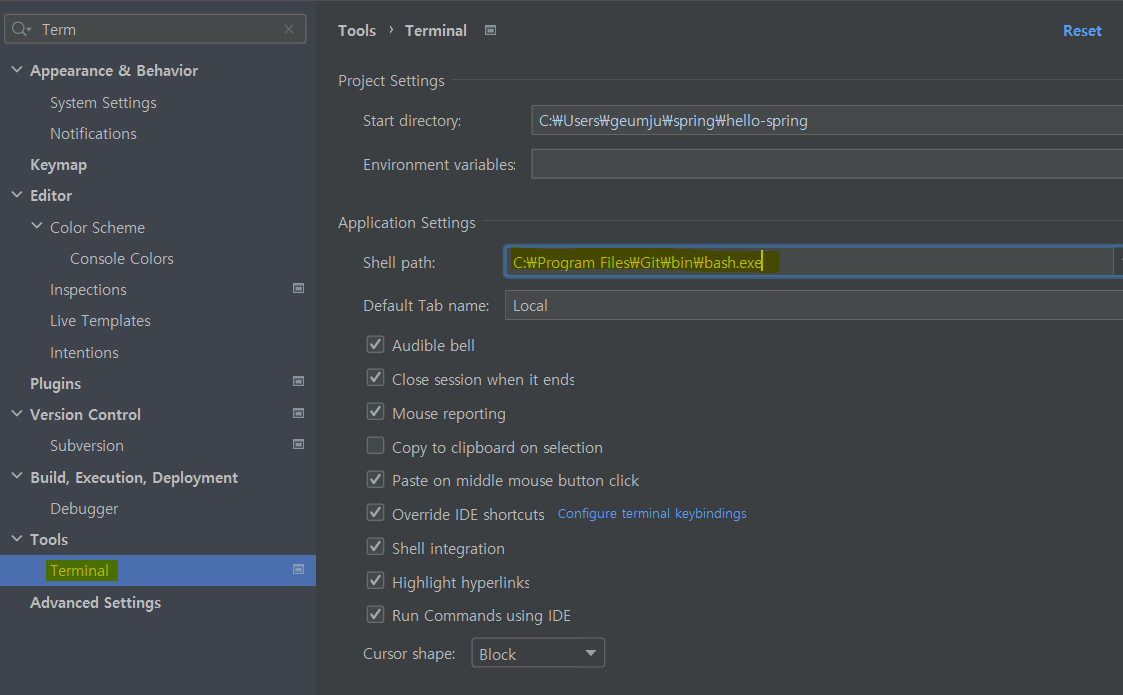
2. 스프링 웹 개발 기초
1. 정적 컨텐츠 : 파일을 그대로 웹 브라우저에게 전달, 프로그래밍 할 수는 없고 원하는 파일을 집어넣으면 웹 브라우저에 그대로 반환
1) 예시로 static에 html형식의 아무 파일이나 만든다

2) html 파일 복붙
<!DOCTYPE HTML>
<html>
<head>
<title>static content</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
</head>
<body>
정적 컨텐츠 입니다.
</body>
</html>
3) 빌드한다

4) 웹브라우저에 localhost:8080/파일명.html로 들어가보기(빌드 상태 유지)
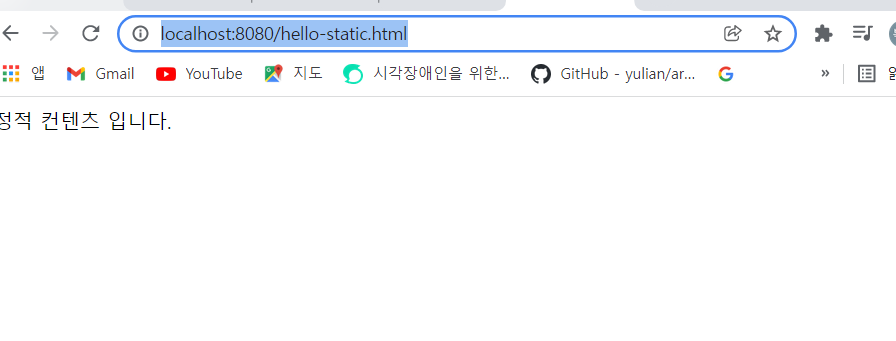
5) 간단하게 표현하자면 컨트롤러가 우선순위를 가져서 1.컨트롤러를 먼저 확인하고 2. resources 안에서 찾아서 반환해줌

2. MVC와 템플릿 엔진 : 서버에서 프로그래밍해서(변형해서) HTML을 동적으로 내려주는 것, 요즘 방식(Model, View, Controller), HTML 방식으로 내림
- 예전에는 view로만 했는데 view는 화면을 그리는데만 집중
- controller나 나머지는 내부처리 및 비즈니스 로직 처리 집중해야 해서 쪼갬
- model에 화면에 관련된 것을 담아서 화면에 넘김
- 프로그래밍 할 때 관심사를 분리해야 함
1) 앞서 이어서 HelloController.java : controller안에 템플릿을 담음[Controller 부분]
package hello.hellospring.control;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
@Controller
public class HelloController {
@GetMapping("hello")
public String hello(Model model){
model.addAttribute("data","hello!");
return "hello";
}
@GetMapping("hello-mvc")
public String helloMvc(@RequestParam("name") String name, Model model){
model.addAttribute("name", name);
return "hello-templates";
}
2) helloMvc에서 리턴하는 hello-templates를 template안에 생성(html로)
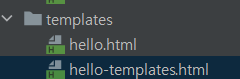
3) hello-templates에 소스를 넣어줌[View 부분]
<html xmlns:th="http://www.thymeleaf.org">
<body>
<p th:text="'hello ' + ${name}">hello! empty</p>
</body>
</html>
: 참고로 실제서버 구현시 "'hello ' + ${name}"에 있는 값이 hello! empty 대신 바뀜 hello! empty은 서버 없이 구현할 때 확인을 위해서 작성
4) ctrl + shift + c(absolute path) 나 hello-templates 소스트리에 오른쪽 마우스 => copy path => absolute path
복사한 것을 빌드없이 열어봐도열린다는 것을 알 수 있음

5) 빌드를 하고 localhost:8080/hello-mvc를 하면 오류가 뜸 (hello-mvc는 자바파일에서 getmapping 옆의 키?)
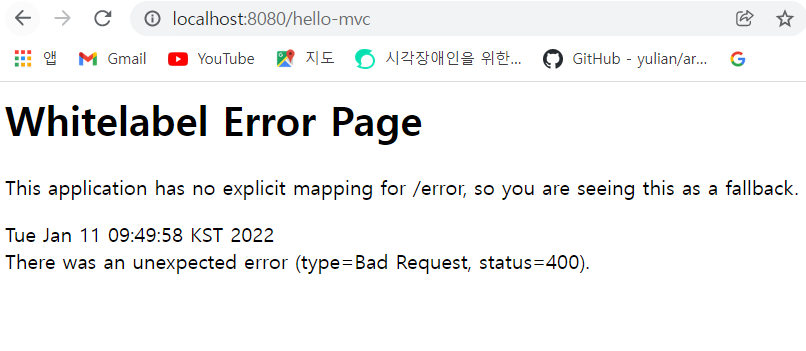
6) 밑에 에러를 읽어보면

7) 파라미터 info를 윈도우/리눅스 사용자는 ctrl + p, 맥 사용자는 cmd + p

8) 기본이 true기 때문에 required는 안적어줘도 됨(false라면 true로 바꿔줘야 함), value도 삭제 가능
즉, 위 코드에서 변경사항 없음(변경할 것이 있는지 확인을 위함)
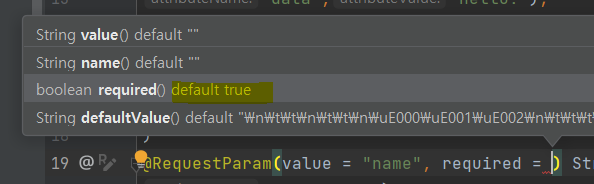
9) http://localhost:8080/hello-mvc?name=spring!!에 들어감

10) 만약 http://localhost:8080/hello-mvc?name=geum로 넣는다면

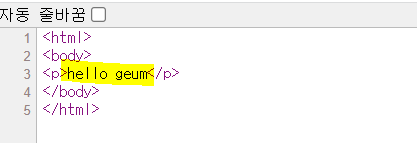
11) 간단한 이미지(키는 name이고 값은 spring으로), viewResolver는 화면과 관련된 해결자가 동작, 변환을 한다는 것, 나한테 맞는 템플릿 찾아서 돌려줘
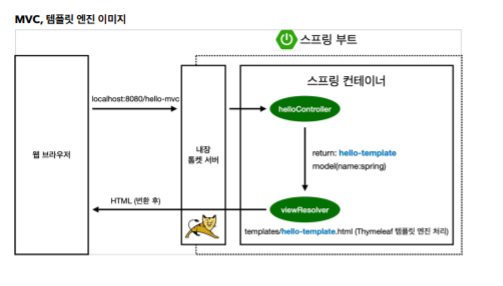
Q. url : localhost:8080/hello-mvc?name=spring은 name은 value에 저장되는 걸까? 아님 변수 name에 저장되는걸까?
A. /hello-mvc?name=spring 요청이 들어오면 controller의 @RequestParam("name") String name(string 타입을 가지면 이름이 name인 변수)로 먼저 spring이 담김 => model.addAttribute의 value로 name 변수를 넘겨줌
즉, controller의 메서드 파라미터로 받은 name 변수를 model에도 넣어줌
3. API : JSON 구조 포맷으로 클라이언트에게 전달, 서버끼리 통신할 때, 리액트, 플루터 등
1) 앞서 이어서 HelloController.java : controller안에 템플릿을 담음(앞이랑 달리 view 이런 거 없이 그냥 내려감
클라이언트에게 그냥 전달됨)
package hello.hellospring.control;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class HelloController {
@GetMapping("hello")
public String hello(Model model){
model.addAttribute("data","hello!");
return "hello";
}
@GetMapping("hello-mvc")
public String helloMvc(@RequestParam("name") String name, Model model){
model.addAttribute("name", name);
return "hello-templates";
}
@GetMapping("hello-string")
@ResponseBody
public String helloString(@RequestParam("name") String name){
return "hello" + name; //"hello spring"
}
}
2) 빌드해준 다음 http://localhost:8080/hello-string?name=spring 들어가면 됨

3) 이 화면의 소스를 보면 딱 문자만 들어와 있음 html 같은 것이 없음(키는 name, value는 spring)
=> 이것은 문자의 경우

4) 데이터의 예시를 보자 java 파일에 소스(객체인 경우) => json 방식으로 넘게 반환하자
- getter와 setter은 프로퍼티방식 private 때문에 사용
package hello.hellospring.control;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class HelloController {
@GetMapping("hello")
public String hello(Model model){
model.addAttribute("data","hello!");
return "hello";
}
@GetMapping("hello-mvc")
public String helloMvc(@RequestParam("name") String name, Model model){
model.addAttribute("name", name);
return "hello-templates";
}
@GetMapping("hello-string")
@ResponseBody
public String helloString(@RequestParam("name") String name){
return "hello " + name; //"hello spring"
}
@GetMapping("hello-api")
@ResponseBody
public Hello helloApi(@RequestParam("name") String name) {
Hello hello = new Hello(); //객체 만들기
hello.setName(name);
return hello; //객체를 넘김
}
static class Hello {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
}
5) 빌드를 하고 http://localhost:8080/hello-api?name=spring에 들어가보면 이렇게 뜸

6) 소스를 보면 아래와 같음 이것은 JSON 방식 JSON 방식은 아래에서 정리

7) @ResponsesBody 사용원리 : 어? ResponsesBody가 있네? 데이터를 그대로 넘겨야 겠구나

@ResponseBody 를 사용
- HTTP의 BODY에 문자 내용을 직접 반환
- viewResolver 대신에 HttpMessageConverter 가 동작
- 기본 문자처리: StringHttpMessageConverter
- 기본 객체처리: MappingJackson2HttpMessageConverter
- byte 처리 등등 기타 여러 HttpMessageConverter가 기본으로 등록되어 있음
3. JSON
1. JavaScript Object Notation (JSON)가 뭘까?
- 구조화된 데이터를 표현하기 위한 문자 기반의 표준 포맷
- KEY : VALUE 구조 => HTML 방식이 무거워서 대신 사용
- 웹 어플리케이션에서 데이터를 전송할 때 일반적으로 사용
- 서버에서 클라이언트로 데이터를 전송하여 표현하려거나 반대의 경우
- SON이 Javascript 객체 문법과 매우 유사하지만 딱히 Javascript가 아니더라도 JSON을 읽고 쓸 수 있는 기능이 다수의 프로그래밍 환경에서 제공
- JSON은 문자열 형태로 존재 네트워크를 통해 전송할 때 아주 유용
- 데이터에 억세스하기 위해서는 네이티브 JSON 객체로 변환될 필요
- 개별 JSON 객체를 .json 확장자를 가진 단순 텍스트 파일에 저장할 수 있다.
2. JSON의 특징
- JSON은 순수히 데이터 포맷입니다. 오직 프로퍼티만 담을 수 있다. 메서드는 담을 수 없음
- JSON은 문자열과 프로퍼티의 이름 작성시 큰 따옴표만을 사용해야 한다. 작은 따옴표는 사용불가
- 콤마나 콜론을 잘못 배치하는 사소한 실수로 인해 JSON파일이 잘못되어 작동하지 않을 수 있다. JSONLint같은 어플리케이션을 사용해 JSON 유효성 검사를 할 수 있다.
- JSON은 JSON내부에 포함할 수 있는 모든 형태의 데이터 타입을 취할 수 있다. 즉, 배열이나 오브젝트 외에도 단일 문자열이나 숫자또한 유효한 JSON 오브젝트가 된다.
- 자바스크립트에서 오브젝트 프로퍼티가 따옴표로 묶이지 않을 수도 있는 것과는 달리, JSON에서는 따옴표로 묶인 문자열만이 프로퍼티로 사용될 수 있다.
3. JSON 구조
JSON는 Javascript 객체 리터럴 문법을 따르는 문자열, 예시: 데이터 계층을 구축가능
{
"squadName": "Super hero squad",
"homeTown": "Metro City",
"formed": 2016,
"secretBase": "Super tower",
"active": true,
"members": [
{
"name": "Molecule Man",
"age": 29,
"secretIdentity": "Dan Jukes",
"powers": [
"Radiation resistance",
"Turning tiny",
"Radiation blast"
]
},
{
"name": "Madame Uppercut",
"age": 39,
"secretIdentity": "Jane Wilson",
"powers": [
"Million tonne punch",
"Damage resistance",
"Superhuman reflexes"
]
},
{
"name": "Eternal Flame",
"age": 1000000,
"secretIdentity": "Unknown",
"powers": [
"Immortality",
"Heat Immunity",
"Inferno",
"Teleportation",
"Interdimensional travel"
]
}
]
}
2) 이 객체를 Javascript 프로그램에서 로드하고, 예를 들어 superHeroes라는 이름의 변수에 파싱하면 점/브라켓 표현법을 통해 객체 내 데이터에 접근할 수 있게 됨
superHeroes.homeTown
superHeroes['active']
3) 하위 계층의 데이터에 접근하려면, 간단하게 프로퍼티 이름과 배열 인덱스의 체인을 통해 접근 예를 들어 superHeroes의 두 번째 member의 세 번째 power에 접근하는 방식은 아래
superHeroes['members'][1]['powers'][2]
- 우선 변수 이름은 — superHeroes
- members 프로퍼티에 접근하려면, ["members"]를 입력
- members는 객체로 구성된 배열입니다. 두 번째 객체에 접근할 것이므로 [1]를 입력
- 이 객체에서 powers 프로퍼티에 접근하려면 ["powers"]를 입력
- powers 프로퍼티 안에는 위에서 선택한 hero의 superpower들이 있다. 세 번째 것을 선택해야 하므로 [2].
4. JSON에서의 배열 : 자바스크립트의 배열 또한 JSON에서 유효
[
{
"name": "Molecule Man",
"age": 29,
"secretIdentity": "Dan Jukes",
"powers": [
"Radiation resistance",
"Turning tiny",
"Radiation blast"
]
},
{
"name": "Madame Uppercut",
"age": 39,
"secretIdentity": "Jane Wilson",
"powers": [
"Million tonne punch",
"Damage resistance",
"Superhuman reflexes"
]
}
]
간단하게 이정도만 일단 알아보고 나머지는 뒤에 가서 더 알아보자
'프레임워크 > 스프링입문' 카테고리의 다른 글
스프링 입문 01편, 웹, JAVA11과 intellJ 설치 및 프로젝트 환경설정 (0) | 2022.01.10 |
---|